Do you want to speed up your Java programs? One powerful way to do this is by using asynchronous database calls. Imagine you’re cooking dinner. You don’t just wait for the vegetables to boil before starting on the rice, right? You multitask! Asynchronous programming works similarly. Instead of waiting for each database query to finish before moving on, your program can handle multiple queries at the same time. This is like having your program “cook” several parts of the meal concurrently, making the whole process much faster and more efficient.
What Are Async Database Calls?
Async database calls allow your application to interact with the database without waiting for a response. Instead of freezing up while waiting for data, your app can handle other tasks. It’s like sending a text and getting notified when there’s a reply — no need to stare at your phone.
- The main thread initiates a database call.
- A callback function handles the response when it arrives.
- Meanwhile, the main thread continues processing other tasks.
Why Use Async Database Calls in Java?
Asynchronous (async) programming has become a cornerstone in modern software development, enabling applications to handle high-concurrency demands efficiently. In Java, async database calls improve application responsiveness by eliminating blocking operations. Instead of waiting for database queries to return results, async programming allows other tasks to continue running, improving both performance and user experience.
Requirements for Asynchronous Programming
Before diving into async programming in Java, ensure that you have
- Basic knowledge of Java threads and concurrency.
- Understanding of Java I/O operations and how blocking I/O differs from non-blocking I/O.
- A database driver that supports async operations, such as R2DBC (Reactive Relational Database Connectivity).
- A suitable Java version (Java 8 or higher) to leverage modern concurrency APIs like CompletableFuture and reactive libraries.
Core Concepts of Async Programming
To implement async database calls effectively, familiarize yourself with these key concepts
- Futures and promises represent the result of an asynchronous computation.
- Non-blocking i/o avoids thread blocking during i/o operations, enabling efficient resource utilization.
- Event-driven programming uses callbacks or event loops to process results once available.
- Reactive streams handles async data streams using publishers, subscribers, and backpressure mechanisms.
Setting Up Your Java Environment
Follow these steps to prepare your environment for async programming
- Install the required jdk ensure you are using java 8 or newer.
- Add dependencies include libraries like R2DBC, Project Reactor, or RxJava in your pom.xml or build.gradle.
- Configure database connectivity set up your database connection properties in a configuration file or environment variables.
- Enable logging use logging frameworks like SLF4J or Logback to monitor async operations.
Using CompletableFuture for Async Calls

The CompletableFuture class in Java simplifies async programming by providing a rich API for managing asynchronous tasks. Here’s an example of using CompletableFuture for async database calls
Integrating Async Database Calls
To integrate async database calls in real-world applications
- Use a thread pool avoid blocking the main thread by delegating database tasks to a thread pool.
- Optimize query performance Ensure database queries are optimized for speed and efficiency.
- Handle timeouts set appropriate timeouts to avoid indefinitely hanging operations.
- Implement retries Use retry mechanisms for transient errors like network issues.
Using Reactive Libraries
Reactive libraries such as Project Reactor and RxJava simplify async programming by providing powerful abstractions. For database operations, R2DBC is a popular choice. Below is an example using R2DBC with Project Reactor

Error Handling in Async Calls
- Use try-catch blocks wrap async code to catch exceptions.
- Handle timeouts use APIs like orTimeout in CompletableFuture to handle delays.
- Log errors record all exceptions and errors for debugging purposes.
- Fallback mechanisms implement fallback operations when async calls fail.
Performance Benefits of Async Programming
Async programming enhances performance in multiple ways
- Better resource utilization avoids blocking threads during I/O operations.
- Improved response times processes multiple requests concurrently without delays.
- Scalability supports a larger number of concurrent users.
Common Pitfalls and How to Avoid Them
Common Pitfalls and How to Avoid Them” is a versatile topic that can be tailored to any field or subject. Here’s a structured approach to explore the concept effectively
Deadlocks
Deadlocks occur when threads are waiting indefinitely for resources held by each other. To prevent deadlocks avoid nested locking minimize the use of nested synchronized blocks.Use timeouts implement timeouts for locks to ensure threads don’t wait indefinitely.Follow a consistent lock acquisition order maintain a strict order for acquiring locks.
Resource Leaks
Failing to release resources like connections can degrade performance. Always close database connections use try-with-resources or explicit close calls. Monitor resource usage leverage tools to track active connections.
Debugging Challenges
Async code can be challenging to debug. Use structured logging provide detailed logs for async operations. Profiling tools monitor thread usage and application performance.
Final Thoughts
Making database calls async in Java can transform your applications, making them faster, more efficient, and capable of handling more users. By understanding Cloud Computing Essentials the core concepts, setting up the right environment, and using tools like CompletableFuture, you’re well on your way to becoming a pro in async programming.
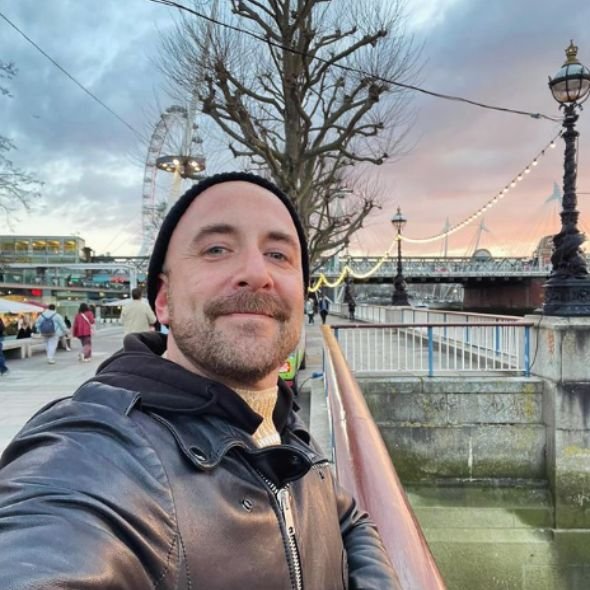